Welcome back! Today’s blog is about how you can create an A.I powered Twitter bot using the well-known python library Tweepy, which will generate tweets on its own without your interaction, is not that amazing? Imagine that you have a bot creating tweets on a topic for you 24 hours even if you are sleeping!
Github: https://github.com/kachkolasa/twitter-bot
Table of Contents
Example
Before we start let’s see some examples of the tweets that the bot generated.
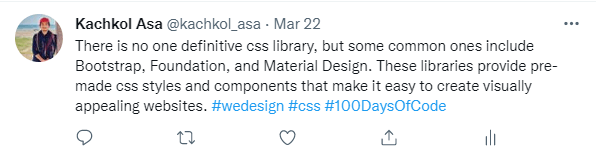
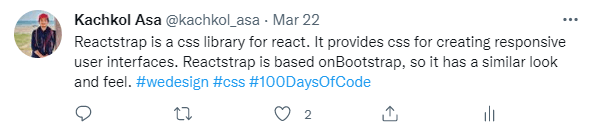
Look at those cool tweets, I even didn’t know about Reactstrap but my bot knows about it. I only told my bot to generate tweets on CSS libraries and it generated them automatically and even tweeted it on its own. I feel like Tony Stark 😉
If you prefer Video then please watch this otherwise you can continue the blog post 🙂
What libraries we will be using?
This Twitter bot was possible because of two free python libraries, Tweepy and OpenAI.
Tweepy: We will be using Tweepy to interact with our Twitter account and publish new tweets. There is a lot of things you can do with Tweepy but for now, we are only going to use it to publish tweets.
You can install Tweepy using this command: pip install tweepy
OpenAI: OpenAI is awesome! We will use OpenAI to generate the tweets, we will only give it the topic or subject that you want to tweet on and it will generate the tweets automatically, here is another fun fact, it can also generate tweets on a hashtag!
You can install OpenAI using this command: pip install openai
Let’s start coding the Twitter bot
Before we start, you will need to get some API keys from the Twitter developer, the keys we need are API Key, API Key Secret, Access Token, and Access Token Secret. You will need to create a project and then an app within that project. Another thing to be remembered is that you will need to upgrade your account from Essential to Elevated so that we can publish tweets using our Twitter bot. And to do that you have to go to Products > Twitter API v2 > Elevated (under the title of the page) and then you will need to fill out a form that one of the developers of Twitter will read and respond to you. They usually say that it takes 48 hours to reach but for me, it was just a few hours.
And also you will need to get the API key for OpenAI, you will need to sign up and then click on the profile icon on the top right corner and then click on view API keys, and then you should see your API key.
Now let’s import the python libraries:
import tweepy, openai, random
As described before OpenAI will generate the tweets and Tweepy will publish them. And the random library will just select a random topic from a list.
Now let’s start TwitterBot() class.
class TwitterBot():
api_key ="YOUR API KEY FROM TWITTER"
api_key_secret ="YOUR API KEY SECRET FROM TWITTER"
access_token="YOUR ACCESS TOKEN FROM TWITTER"
access_token_secret="YOUR ACCESS TOKEN SECRET FROM TWITTER"
#OpenAI
openai.api_key = "OPEN AI KEY"
In the above codes, we are just creating a class naming it TwitterBot(), and then we are just assigning some values to api_key, api_key_secret, access_token, access_token_secret variables. The next thing we did is setting up the API key for OpenAI using the openai.api_key method.
Now let’s connect with the Tweepy library
class TwitterBot():
api_key ="YOUR API KEY FROM TWITTER"
api_key_secret ="YOUR API KEY SECRET FROM TWITTER"
access_token="YOUR ACCESS TOKEN FROM TWITTER"
access_token_secret="YOUR ACCESS TOKEN SECRET FROM TWITTER"
#OpenAI
openai.api_key = "OPEN AI KEY"
# Connecting to Twitter
auth = tweepy.OAuthHandler(api_key, api_key_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit=True)
The codes added to the bottom are connecting with Twitter using the Tweepy library.
class TwitterBot():
api_key ="YOUR API KEY FROM TWITTER"
api_key_secret ="YOUR API KEY SECRET FROM TWITTER"
access_token="YOUR ACCESS TOKEN FROM TWITTER"
access_token_secret="YOUR ACCESS TOKEN SECRET FROM TWITTER"
#OpenAI
openai.api_key = "OPEN AI KEY"
# Connecting to Twitter
auth = tweepy.OAuthHandler(api_key, api_key_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit=True)
# Creating Tweets
prompts = [
{
"hashtag": "#meme",
"text": "post a meme on twitter bots"
},
{
"hashtag": "#technology",
"text": "tweet something cool for #technology"
},
{
"hashtag": "@kachkol_asa",
"text": "tweet something cool for @kachkol_asa from twitter"
},
]
Now we are creating the prompts for OpenAI or you can say the topics, and also we are adding some hashtags. You can add yours as well, of course, just change the hashtag and text or add new ones to the list.
class TwitterBot():
api_key ="YOUR API KEY FROM TWITTER"
api_key_secret ="YOUR API KEY SECRET FROM TWITTER"
access_token="YOUR ACCESS TOKEN FROM TWITTER"
access_token_secret="YOUR ACCESS TOKEN SECRET FROM TWITTER"
#OpenAI
openai.api_key = "OPEN AI KEY"
# Connecting to Twitter
auth = tweepy.OAuthHandler(api_key, api_key_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit=True)
# Creating Tweets
prompts = [
{
"hashtag": "#meme",
"text": "post a meme on twitter bots"
},
{
"hashtag": "#technology",
"text": "tweet something cool for #technology"
},
{
"hashtag": "@kachkol_asa",
"text": "tweet something cool for @kachkol_asa from twitter"
},
]
def __init__(self):
error = 1
while(error == 1):
tweet = self.create_tweet()
try:
error = 0
self.api.update_status(tweet)
except:
error = 1
Now let’s define the __init__ method, what this method is doing is that it generate a tweet using the create_tweet() method (which we need to define later), and then publishing the tweet using the update_status() method in Tweepy. It also checks if there was an error in the process like if the text was so much then it will generate another one.
class TwitterBot():
api_key ="YOUR API KEY FROM TWITTER"
api_key_secret ="YOUR API KEY SECRET FROM TWITTER"
access_token="YOUR ACCESS TOKEN FROM TWITTER"
access_token_secret="YOUR ACCESS TOKEN SECRET FROM TWITTER"
#OpenAI
openai.api_key = "OPEN AI KEY"
# Connecting to Twitter
auth = tweepy.OAuthHandler(api_key, api_key_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit=True)
# Creating Tweets
prompts = [
{
"hashtag": "#meme",
"text": "post a meme on twitter bots"
},
{
"hashtag": "#technology",
"text": "tweet something cool for #technology"
},
{
"hashtag": "@kachkol_asa",
"text": "tweet something cool for @kachkol_asa from twitter"
},
]
def __init__(self):
error = 1
while(error == 1):
tweet = self.create_tweet()
try:
error = 0
self.api.update_status(tweet)
except:
error = 1
def create_tweet(self):
chosen_prompt = random.choice(self.prompts)
text = chosen_prompt["text"]
hashtags = chosen_prompt["hashtag"]
response = openai.Completion.create(
engine="text-davinci-001",
prompt=text,
max_tokens=64,
)
tweet = response.choices[0].text
tweet = tweet + " " + hashtags
return
Now comes the most interesting part, generating the tweets using A.I. The create_tweet() method is randomly selecting a topic from the prompts list and then creates some text (tweet). That is possible because of OpenAI, we are using the text-davinci-001 engine to generate the tweet, there are much more engines and other parameters in OpenAI, I strongly suggest checking on OpenAI Playground.
Now we have an A.I powered Twitter bot ready, we only need to run the TwitterBot() class, and boom, it will do all the things on its own. Here are the final codes:
import openai, tweepy, random
class TwitterBot():
api_key ="YOUR API KEY FROM TWITTER"
api_key_secret ="YOUR API KEY SECRET FROM TWITTER"
access_token="YOUR ACCESS TOKEN FROM TWITTER"
access_token_secret="YOUR ACCESS TOKEN SECRET FROM TWITTER"
#OpenAI
openai.api_key = "OPEN AI KEY"
# Connecting to Twitter
auth = tweepy.OAuthHandler(api_key, api_key_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit=True)
# Creating Tweets
prompts = [
{
"hashtag": "#meme",
"text": "post a meme on twitter bots"
},
{
"hashtag": "#technology",
"text": "tweet something cool for #technology"
},
{
"hashtag": "@kachkol_asa",
"text": "tweet something cool for @kachkol_asa from twitter"
},
]
def __init__(self):
error = 1
while(error == 1):
tweet = self.create_tweet()
try:
error = 0
self.api.update_status(tweet)
except:
error = 1
def create_tweet(self):
chosen_prompt = random.choice(self.prompts)
text = chosen_prompt["text"]
hashtags = chosen_prompt["hashtag"]
response = openai.Completion.create(
engine="text-davinci-001",
prompt=text,
max_tokens=64,
)
tweet = response.choices[0].text
tweet = tweet + " " + hashtags
return tweet
twitter = TwitterBot()
twitter.create_tweet()
Thanks for reading this article, you can also read: How to make money as a web developer and How to become a web developer without any degree.
1 thought on “How to build an A.I powered Twitter Bot using Tweepy python library 2022”